Natural Language Processing with Disaster Tweetsrに関する4回目の記事です。
今回はデータクレンジングを行います。
学習データと検証データの結合
まずは一括でデータクレンジングするために、学習データと検証データを結合します。
[ソース]
1 | df = pd.concat([tweet,test]) |

URLの排除
URLの排除を行います。正規表現を使ってURLパターンに合致したものを排除します。
[ソース]
1 | example = "New competition launched :https://www.kaggle.com/c/nlp-getting-started" |
[結果]

HTMLタグの排除
HTMLタグも正規表現を使って排除します。
[ソース]
1 | example = """<div> |
[結果]
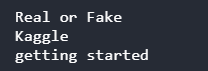
絵文字の排除
絵文字も正規表現を使って排除します。
複数パターンある場合は、リスト型を使ってまとめて指定することができます。
[ソース]
1 | # Reference : https://gist.github.com/slowkow/7a7f61f495e3dbb7e3d767f97bd7304b |
[結果]

句読点の排除
句読点を排除します。下記の方法で文字の変換を行っています。
- str.maketrans()でstr.translate()に使える変換テーブルを作成する。
- str.translate()で文字列内の文字を変換する。
[ソース]
1 | def remove_punct(text): |
[結果]

スペル修正
最後にスペルの修正を行います。
pyspellcheckerというライブラリを使うので、まずこれをインストールしておきます。
[コマンド]
1 | !pip install pyspellchecker |
[結果]

スペルチャックは一旦単語ごとに分解し、スペルミスがあれば正しいスペルに修正し、最後に修正した単語を含めて結合し直しています。
[ソース]
1 | from spellchecker import SpellChecker |
[結果]

今回は、英語文字列のクレンジングを行いました。
次回は、単語ベクター化モデルの一つであるGloVeを試してみます。