レーストラック最適化
レーストラック最適化問題では、車両が最短時間でコースを周回するための最適な制御を見つけることが目的です。
この問題は、CVXPYを使用して凸最適化問題として定式化できます。
以下に、簡単な例を示します。
問題設定:
🔹車両は、一定の最大加速度と最大速度を持っています。
🔹車両は、所定のスタート地点から始まり、所定の終了地点で終わります。
🔹車両は、所定の制約条件下で最短時間でコースを周回することが目的です。
解法
CVXPYを使用して上記の問題を解いていきます。
まず、必要なライブラリをインポートします。
1 2
| import cvxpy as cp import numpy as np
|
次に、問題のパラメータを定義します。
1 2 3 4 5
| T = 100 dt = 0.1 v_max = 5.0 a_max = 2.0
|
状態変数と制御変数を定義します。
1 2 3 4
| x = cp.Variable((T, 2)) v = cp.Variable((T, 2)) a = cp.Variable((T - 1, 2))
|
制約条件を定義します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| constraints = []
constraints += [x[0] == np.array([0, 0])] constraints += [v[0] == np.array([0, 0])]
constraints += [x[-1] == np.array([10, 10])]
for t in range(T - 1): constraints += [x[t + 1] == x[t] + dt * v[t]] constraints += [v[t + 1] == v[t] + dt * a[t]]
constraints += [cp.norm(v, axis=1) <= v_max]
constraints += [cp.norm(a, axis=1) <= a_max]
|
目的関数を定義し、問題を解きます。
1 2 3 4 5 6 7 8
| objective = cp.Minimize(dt * cp.sum(cp.norm(v, axis=1)))
problem = cp.Problem(objective, constraints)
result = problem.solve()
|
この例では、車両は所定のスタート地点から始まり、所定の終了地点で終わり、最大速度と最大加速度の制約の下で最短時間でコースを周回することが目的です。
CVXPYを使用して、この問題を凸最適化問題として定式化し、最適な制御を見つけることができます。
結果表示
最適化が完了したら、以下のようにして結果を表示できます。
1. 最適な総移動時間を表示する:
1
| print("Optimal total travel time:", result)
|
[実行結果]

2. 最適な軌道を表示する:
1 2 3 4 5 6 7 8 9 10 11
| import matplotlib.pyplot as plt
x_values = x.value[:, 0] y_values = x.value[:, 1]
plt.plot(x_values, y_values, marker='o') plt.xlabel('x') plt.ylabel('y') plt.title('Optimal Trajectory') plt.grid(True) plt.show()
|
[実行結果]
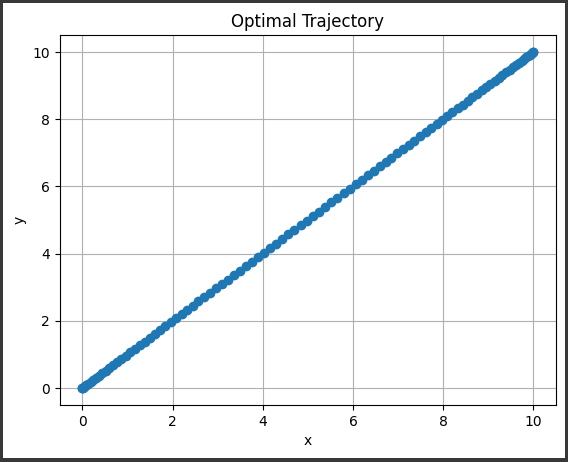
3. 最適な速度プロファイルを表示する:
1 2 3 4 5 6 7 8
| time_steps = np.arange(T) * dt
plt.plot(time_steps, np.linalg.norm(v.value, axis=1)) plt.xlabel('Time (s)') plt.ylabel('Speed (m/s)') plt.title('Optimal Speed Profile') plt.grid(True) plt.show()
|
このコードは、最適な速度プロファイルをプロットし、時間に対する車両の速度を表示します。
[実行結果]
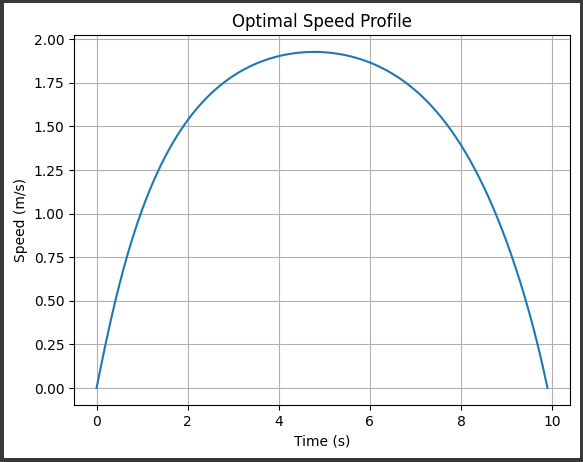